You're browsing the documentation for v2.x and earlier. For v3.x, click here.
Vue has support for slots built in and it is based on the shadow dom. It is a specification that is still in development but worth taking a look at to learn more about how they work, should this development approach become more common. Note: If there is no slotslot element in the child's template, any content from the parent will be silently discarded. Fallback Content. If the parent does not inject any content into the child's slot, the child will render any elements inside its slotslot tag, like so.
This page assumes you've already read the Components Basics. Read that first if you are new to components.
keep-alive
with Dynamic Components
Earlier, we used the is
attribute to switch between components in a tabbed interface:
When switching between these components though, you'll sometimes want to maintain their state or avoid re-rendering for performance reasons. For example, when expanding our tabbed interface a little:
You'll notice that if you select a post, switch to the Archive tab, then switch back to Posts, it's no longer showing the post you selected. That's because each time you switch to a new tab, Vue creates a new instance of the currentTabComponent
.
Recreating dynamic components is normally useful behavior, but in this case, we'd really like those tab component instances to be cached once they're created for the first time. To solve this problem, we can wrap our dynamic component with a element:
Check out the result below:
Now the Posts tab maintains its state (the selected post) even when it's not rendered. See this example for the complete code.
Note that requires the components being switched between to all have names, either using the
name
option on a component, or through local/global registration.
Check out more details on in the API reference.
Async Components
In large applications, we may need to divide the app into smaller chunks and only load a component from the server when it's needed. To make that easier, Vue allows you to define your component as a factory function that asynchronously resolves your component definition. Vue will only trigger the factory function when the component needs to be rendered and will cache the result for future re-renders. For example:
As you can see, the factory function receives a resolve
callback, which should be called when you have retrieved your component definition from the server. You can also call reject(reason)
to indicate the load has failed. The setTimeout
here is for demonstration; how to retrieve the component is up to you. One recommended approach is to use async components together with Webpack's code-splitting feature:
/78059095-56a741e83df78cf77293bdb9.jpg)
You can also return a Promise
in the factory function, so with Webpack 2 and ES2015 syntax you can make use of dynamic imports:
When using local registration, you can also directly provide a function that returns a Promise
:
Vuejs Dynamic Slots Games
If you're a Browserify user that would like to use async components, its creator has unfortunately made it clear that async loading 'is not something that Browserify will ever support.' Officially, at least. The Browserify community has found some workarounds, which may be helpful for existing and complex applications. For all other scenarios, we recommend using Webpack for built-in, first-class async support.
Handling Loading State
New in 2.3.0+
The async component factory can also return an object of the following format:
Note that you must use Vue Router 2.4.0+ if you wish to use the above syntax for route components.
Vue 2.6 is released with new syntax for Slots using v-slot
directive. In this tutorial, we're gonna show you:
- Syntax to use Vue Slot with
v-slot
directive along with its shorthand - How to use Vue Named Slots with
v-slot
& examples - How to use Vue
v-slot
for Scoped Slots & examples - Vue Dynamic slots example

Related Post: Vue 3 Composition API tutorial with examples
Contents
Vue slots syntax with v-slot directive
With new v-slot
directive, we can:
– combine html layers: component tag and scope of the slot.
– combine the slot and the scoped slot in a single directive.
For example, this is old syntax with slot-scope
:
This is how we combine ListComponent
and template
tag:
And this is old named slots syntax:
Now we use new Vue v-slot
directive:
You can see that:
– We use to wrap
tag instead of
directly. This is because Vue v-slot
can only be used in or
html tag. It cannot be used in plain HTML tags (
for example).
– We replace slot='content' slot-scope='{data}'
with v-slot:content='{data}'
by combining slot
& slot-scope
. With new Vue v-slot
directive, all slots are compiled into scoped slots. It improves the performance. Why?
Normal slots are rendered during the parent component's render cycle. So, if any dependency of a slot changes, both the parent and child components will be re-rendered.
When we use scoped slots, slots are compiled into inline functions and called during the child component's render cycle. This means:
- data from a scoped slot are collected by the child component which is re-rendered separately.
- the changes of parent scope dependency only affect the parent, not the child component. So the child component doesn't need to update if it uses only scoped slots.
Shorthand for v-slot
#
is the shorthand for Vue v-slot
directive.
For example, #content
stands for v-slot:content
.
The code above can be written as:
Remember that when using shorthand, we must always specify the name of the slot after #
symbol. We cannot use shorthand like this: #='{item}'
.
It must be: #default='{item}'
in which, #default
is the shorthand for v-slot:default
.
In the next parts, we show you some examples that apply new Vue v-slot
directive in practice.
Vue v-slot examples with Named Slots
If we want to use multiple slots in one component, Named Slots are useful.
The code below shows BkrCard
component template with 3 slots:
Vuejs Dynamic Slots Definition
- header
- title
- default
Remember that without
name
attribute has the name default
.
Vuejs Dynamic Slots Game
Now look at the parent component which use v-slot
directive to specify name for named slots on tag:
The result will be:
If we pass only one named slot, the default value will be shown:
Vue v-slot example with default slot
In the example above, we use for the default slot.
We have other ways to specify html code to be considered as default slot also:
– wrap it in a without Vue
v-slot
directive:
– do not wrap it in a :
The result are the same for 2 cases:
Vue v-slot examples with Scoped Slots
What we should do when we want a child component to allow parent component access its data?
Vuejs Dynamic Slot Content
In this example, categories
need to be available to the slot content in the parent. So we bind the categories
as an attribute to the element:
The categories
attribute is called slot props.
In the parent scope, Vue v-slot
directive can help us get value of the slot props above:
The result will be:
- Dart
- Flutter
- Vue.js
This is shorthand for v-slot
:
Vue Dynamic slots example
We can use a JavaScript expression in v-slot
directive argument with square brackets:
Now look at the example:
Clicking on the Change button will change the collection
value dynamically.v-slot:[collection]='{categories}'
could become:
v-slot:default='{categories}'
v-slot:new_categories='{categories}'
This is BkrCategories
component with default
and new_categories
slot name:
The result will be:
Conclusion
We've learned almost aspects of new Vue v-slot
directive, from v-slot
syntax to its handshort, then apply v-slot
directive on Named Slot examples to Scoped Slots and Dynamic Slots examples.
Happy learning! See you again!
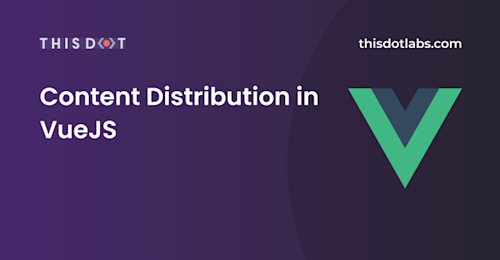
You can also return a Promise
in the factory function, so with Webpack 2 and ES2015 syntax you can make use of dynamic imports:
When using local registration, you can also directly provide a function that returns a Promise
:
Vuejs Dynamic Slots Games
If you're a Browserify user that would like to use async components, its creator has unfortunately made it clear that async loading 'is not something that Browserify will ever support.' Officially, at least. The Browserify community has found some workarounds, which may be helpful for existing and complex applications. For all other scenarios, we recommend using Webpack for built-in, first-class async support.
Handling Loading State
New in 2.3.0+
The async component factory can also return an object of the following format:
Note that you must use Vue Router 2.4.0+ if you wish to use the above syntax for route components.
Vue 2.6 is released with new syntax for Slots using v-slot
directive. In this tutorial, we're gonna show you:
- Syntax to use Vue Slot with
v-slot
directive along with its shorthand - How to use Vue Named Slots with
v-slot
& examples - How to use Vue
v-slot
for Scoped Slots & examples - Vue Dynamic slots example
Related Post: Vue 3 Composition API tutorial with examples
Contents
Vue slots syntax with v-slot directive
With new v-slot
directive, we can:
– combine html layers: component tag and scope of the slot.
– combine the slot and the scoped slot in a single directive.
For example, this is old syntax with slot-scope
:
This is how we combine ListComponent
and template
tag:
And this is old named slots syntax:
Now we use new Vue v-slot
directive:
You can see that:
– We use to wrap
tag instead of
directly. This is because Vue v-slot
can only be used in or
html tag. It cannot be used in plain HTML tags (
for example).
– We replace slot='content' slot-scope='{data}'
with v-slot:content='{data}'
by combining slot
& slot-scope
. With new Vue v-slot
directive, all slots are compiled into scoped slots. It improves the performance. Why?
Normal slots are rendered during the parent component's render cycle. So, if any dependency of a slot changes, both the parent and child components will be re-rendered.
When we use scoped slots, slots are compiled into inline functions and called during the child component's render cycle. This means:
- data from a scoped slot are collected by the child component which is re-rendered separately.
- the changes of parent scope dependency only affect the parent, not the child component. So the child component doesn't need to update if it uses only scoped slots.
Shorthand for v-slot
#
is the shorthand for Vue v-slot
directive.
For example, #content
stands for v-slot:content
.
The code above can be written as:
Remember that when using shorthand, we must always specify the name of the slot after #
symbol. We cannot use shorthand like this: #='{item}'
.
It must be: #default='{item}'
in which, #default
is the shorthand for v-slot:default
.
In the next parts, we show you some examples that apply new Vue v-slot
directive in practice.
Vue v-slot examples with Named Slots
If we want to use multiple slots in one component, Named Slots are useful.
The code below shows BkrCard
component template with 3 slots:
Vuejs Dynamic Slots Definition
- header
- title
- default
Remember that without
name
attribute has the name default
.
Vuejs Dynamic Slots Game
Now look at the parent component which use v-slot
directive to specify name for named slots on tag:
The result will be:
If we pass only one named slot, the default value will be shown:
Vue v-slot example with default slot
In the example above, we use for the default slot.
We have other ways to specify html code to be considered as default slot also:
– wrap it in a without Vue
v-slot
directive:
– do not wrap it in a :
The result are the same for 2 cases:
Vue v-slot examples with Scoped Slots
What we should do when we want a child component to allow parent component access its data?
Vuejs Dynamic Slot Content
In this example, categories
need to be available to the slot content in the parent. So we bind the categories
as an attribute to the element:
The categories
attribute is called slot props.
In the parent scope, Vue v-slot
directive can help us get value of the slot props above:
The result will be:
- Dart
- Flutter
- Vue.js
This is shorthand for v-slot
:
Vue Dynamic slots example
We can use a JavaScript expression in v-slot
directive argument with square brackets:
Now look at the example:
Clicking on the Change button will change the collection
value dynamically.v-slot:[collection]='{categories}'
could become:
v-slot:default='{categories}'
v-slot:new_categories='{categories}'
This is BkrCategories
component with default
and new_categories
slot name:
The result will be:
Conclusion
We've learned almost aspects of new Vue v-slot
directive, from v-slot
syntax to its handshort, then apply v-slot
directive on Named Slot examples to Scoped Slots and Dynamic Slots examples.
Happy learning! See you again!